Retrieving Assets from Installed Asset Packs
Once you have downloaded the Asset Pack, you will need to retrieve the Assets from the pack in order to use them. I have created a lightweight, intelligent, easy to use blueprint node that can fetch the asset for you from the mounted Asset Pack.
There are two primary Asset types that need to be used explicitly and that is Classes and Objects. Classes can be, but are not limited to blueprints, which can be used for spawning or creating things. Objects can be, but are definitely not limited to Textures.
I have put a great deal of effort to reduce the complexities required to identify which is which and make it as easy to use as possible. That being said, it is important you understand how this works as there are always cases in which you may need to handle things according to your situation.
This functionality also identifies when its being run in the Unreal Engine editor and will automatically return what was passed in, instead of attempting to retrieve it from a non-existant Asset Pack. This will allow you to test your functionality as per normal in the Editor.
Blueprint Description
Lets take a high level look at the blueprint itself and its parameter and then provide a slightly more detailed look into how to use it in some examples.
Get Packed Asset
This blueprint node retrieves an Asset from any downloaded Asset Pack.
NOTE: If the Asset does not exist, it will safely return null pointers along with an error message.
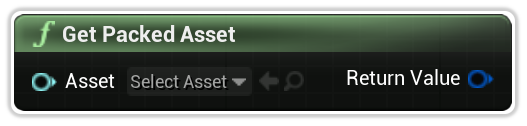
Asset
The Asset you would like retrieved.
Return Value
Returns a Packed Asset struct containing your asset.
Common Examples
Retrieving a Class Asset
Class type assets, such as blueprints or user widgets stored in Asset Packs can easily retrieved at runtime, provided your Asset Pack has been downloaded and installed. Behind the scenes, the Return Value contains an Object pointer and a Class Type pointer. For Class Assets, the Class Type pointer is used.
Below we have a few examples of using the Asset as Class type Asset.
Simply select the BP of the actor you would like fetched from a downloaded Asset Pack as the input. Drag the Return Value onto the Class Input field of the Spawn Actor blueprint node and it will automatically cast it for you.
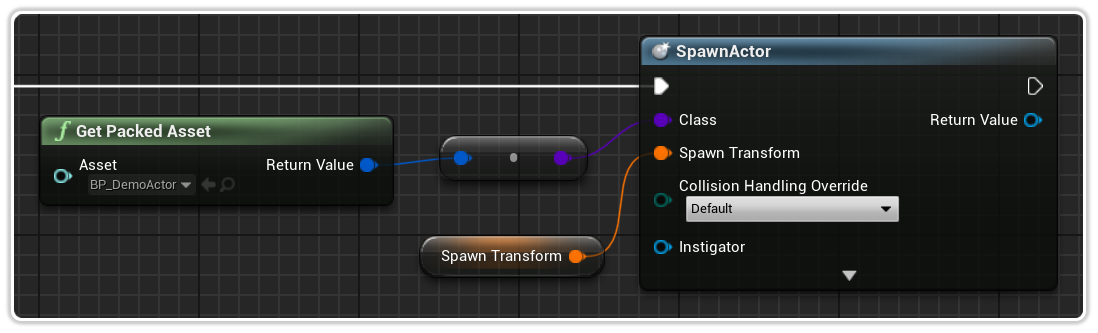
Simply select the Widget BP you would like fetched from a downloaded Asset Pack as the input. Drag the Return Value onto the Class Input field of the Create User Widget blueprint node and it will automatically cast it for you.
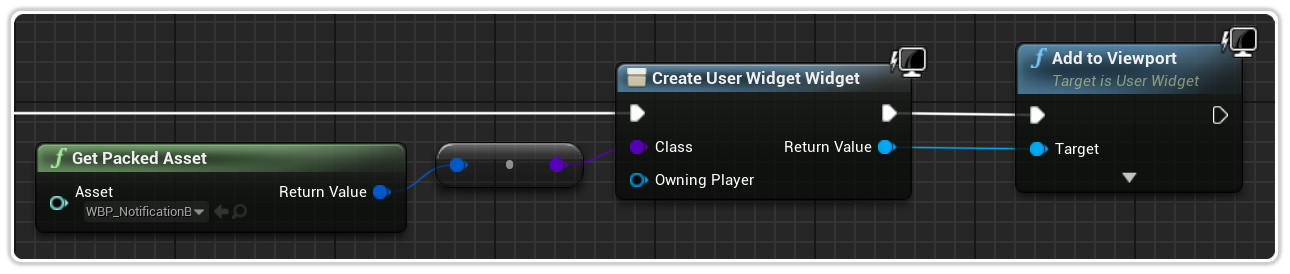
In the situation where the plugin does not have an automatic cast for you, you can simply cast it yourself as long as the type you are casting to is not within an Asset Pack (more on that below).
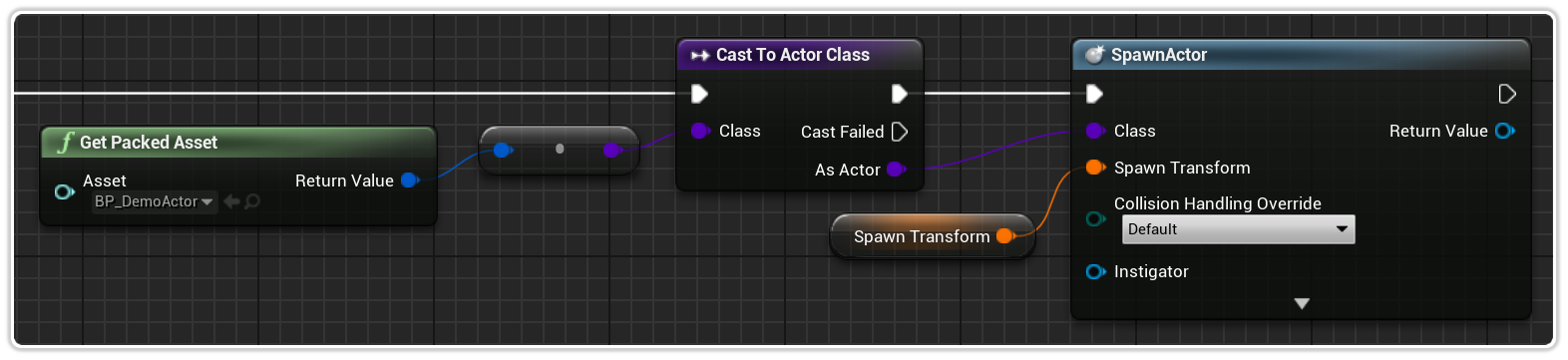
Using Packed Blueprint Functions
If you have loaded a blueprint from an Asset Pack and want to use its function calls, Unreal Engine will not know how to cast it to that type, as that type is unknown to Unreal Engine. In the editor, it will allow you to use a "Cast to [ClassType]" blueprint node, however, that node is entirely generated before you have installed the Class.
What this means is that if you would like to cast a blueprint to a specific type in order to call its functions, the cast will fail.
The way around this is to create a Blueprint Interface, which simply is a place where you define your functions (not the implementation of them). Then, in the blueprint you want to have these functions, you can add this interface and implement the corresponding functions of the interface in your blueprint.
Ultimately, instead of relying on the blueprint as the type, you rely on the interface as the type.
Simply right click anywhere in your Content Browser to bring up the below menu. Select Blueprints and Blueprint Interface.
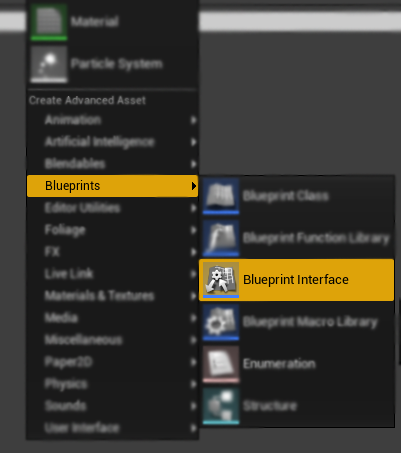
Name your Blueprint Interface and double click it to open it. Here you can create functions and define their input and outputs.
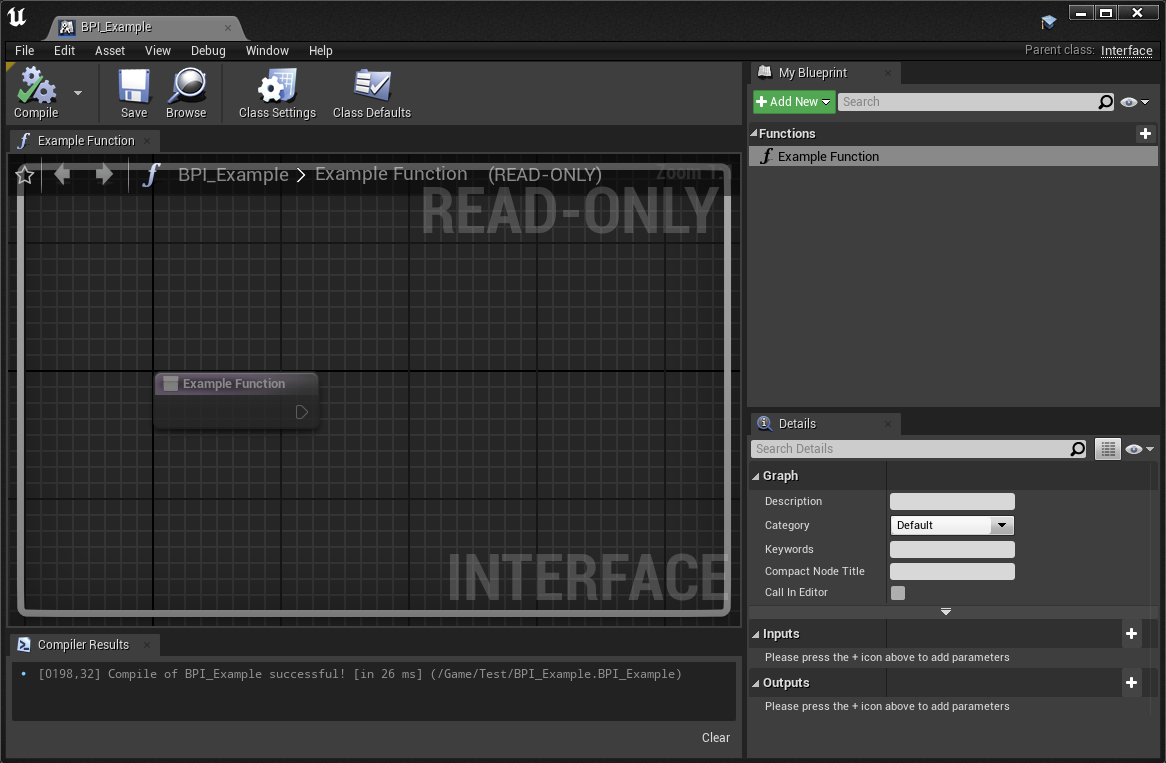
In your Blueprint, go to Class Setting and in the details panel add your Blueprint Interface.
Your Interface will appear on the right, right click it and click Implement Event. Now you can implement what you want your function to do.
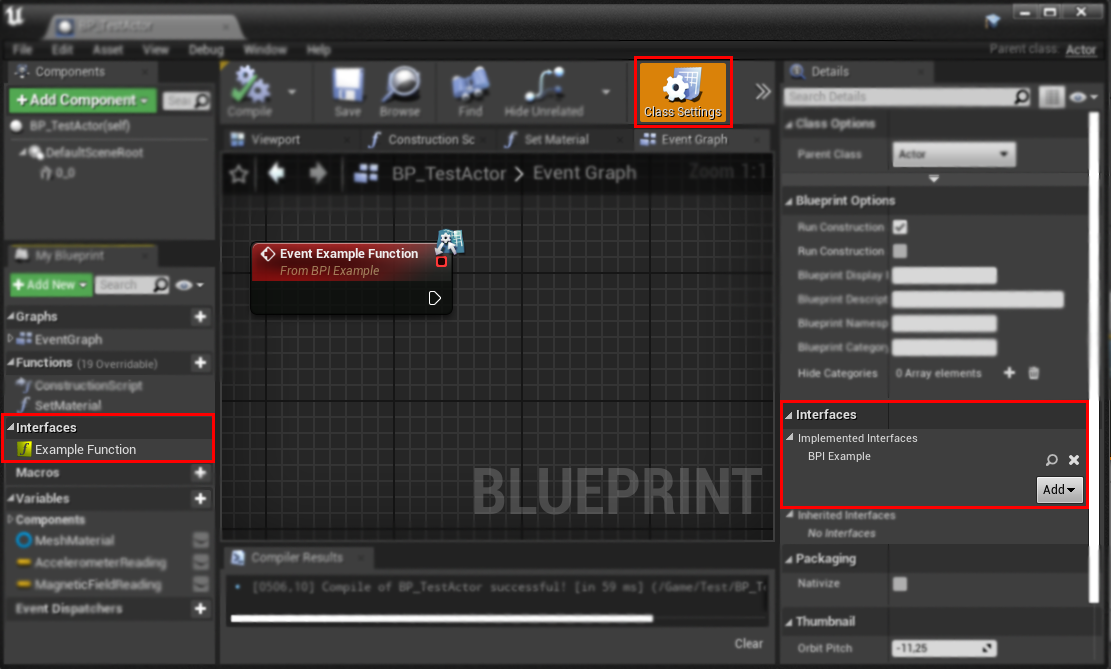
In this example, we are Spawning our Actor, Casting it to our Interface and then calling the interface method Set Material using a Material we also fetched from an Asset Pack.
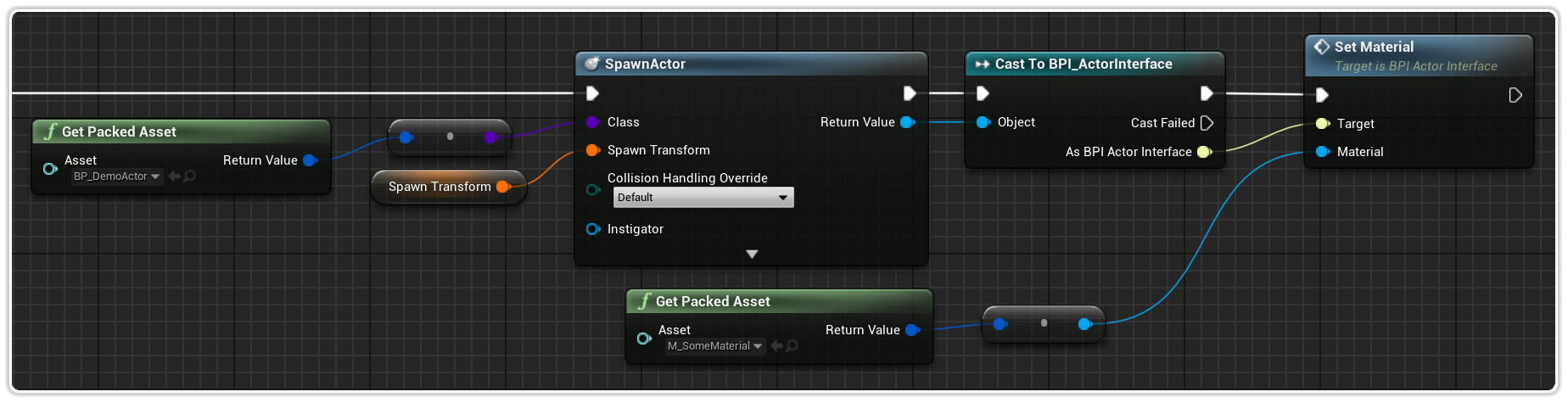
Leave the Blueprint Interface out of any chunks so that Unreal Engine always knows about it at compile time. When you want to call your blueprint's functions, you can cast the Asset to the interface and call the functions as per normal.
Retrieving an Object Asset
Object type assets, such as a Material or Texture2D which are stored in Asset Packs can easily retrieved at runtime, provided your Asset Pack has been downloaded and installed. Behind the scenes, the Return Value contains an Object pointer and a Class Type pointer. For Object Assets, the Object pointer is used.
Below we have a few examples of using the Asset as an Object type Asset.
Simply select the Texture you would like fetched from a downloaded Asset Pack as the input. The Return Value may automatically cast to a Texture2D for you.
In this example, we are using Textures as Texture2D assets for our Android Notification Plugin's blueprint node.
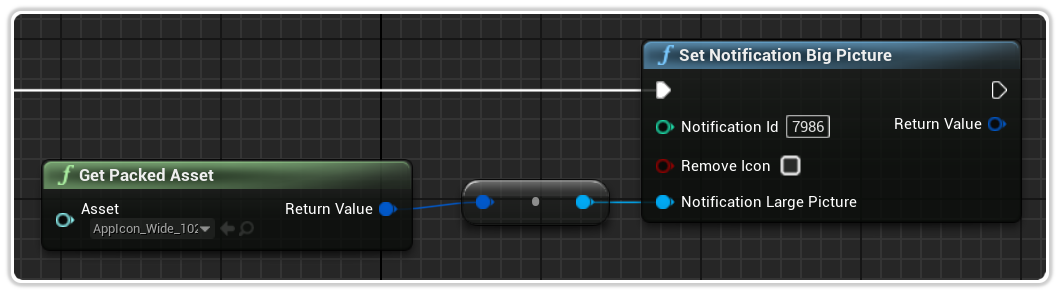
Simply seleect the Material you would like fetched from a downloaded Asset Pack as the input. Be aware that dragging the Return Value onto the Material Input field may not always cast the type for you automatically.
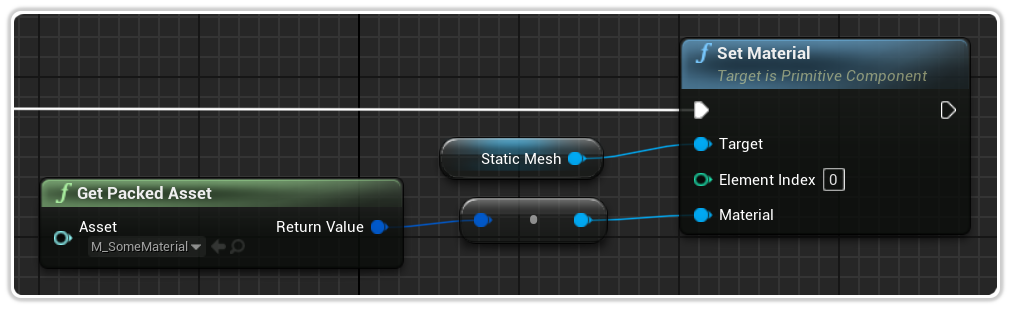
In the situation where the plugin does not have a predefined cast for you, or it does not automatically get picked up, you can simply cast it yourself.
Here we are splitting the Return Value struct for further transparency.
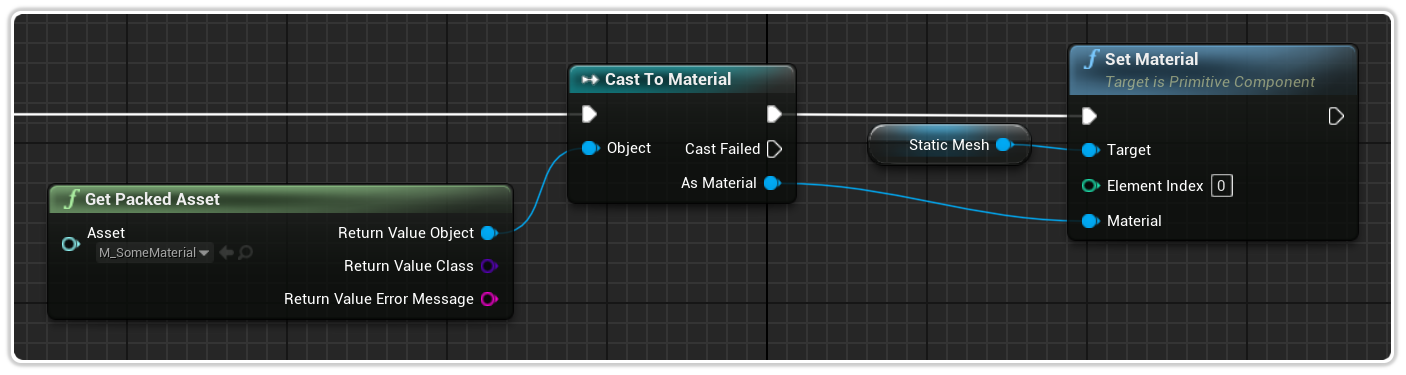
NOTE: Object automatic casting does not always pop up automatically for you when you drag and drop onto another pin. You may need to search for the cast if you do not want to cast it yourself.
List of Automatic Conversions
I have implemented a bunch of automatic conversions for you so that you can simply drag and drop the result of the blueprint node to where you want to use it.
The list is not exhaustive and certainly will never contain your custom types. However, if you do want some conversions added to this list, please feel free to reach out and I can look into adding them to simplify your blueprint setup.
To see the current list, you can simply drag the result of this blueprint node and it will show the full list.
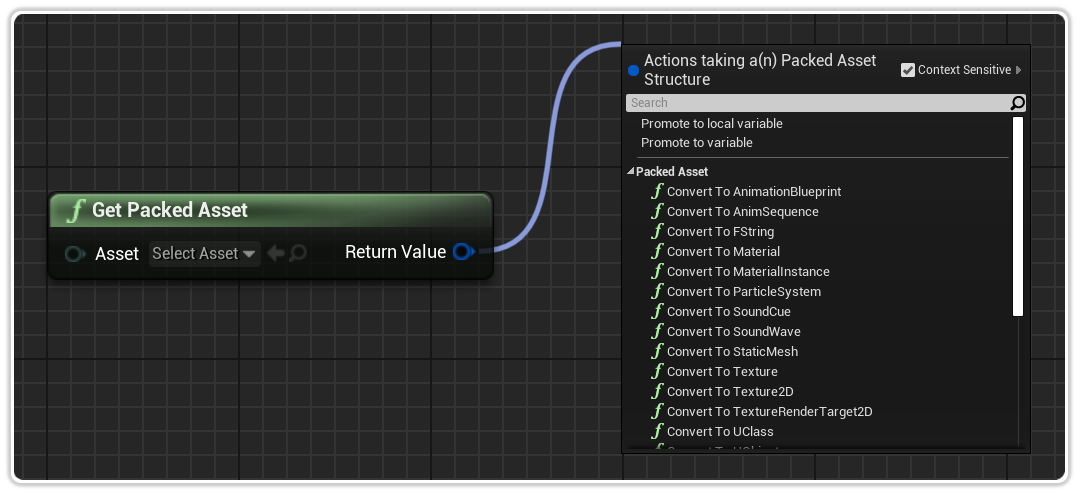